All about React Guideline to become a Web Developer
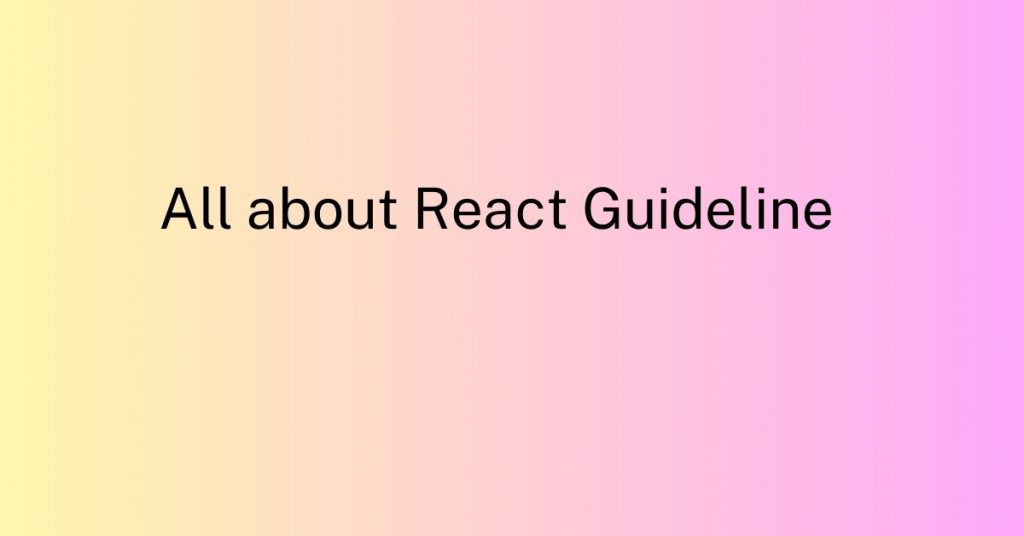
React.js is a popular JavaScript library used for building user interfaces, particularly for single-page applications (SPAs). Here’s a set of guidelines to help you get started with React.js:
1 Prerequisites:
- Basic understanding of HTML, CSS, and JavaScript.
- Familiarity with modern JavaScript features (ES6+).
- Knowledge of Node.js and npm (Node Package Manager) is helpful for setting up React projects.
2 Setting up a React project:
- You can create a new React project using Create React App (CRA) or other build tools like webpack and Babel.
- Use CRA for quick setup:
npx create-react-app my-app
ornpm init react-app my-app
. - Navigate to the project directory:
cd my-app
.
3 Components:
- React is all about components. A component is a reusable and self-contained piece of UI.
- Create components as functional components using
function MyComponent() { ... }
or class components usingclass MyComponent extends React.Component { ... }
. - Components should return JSX (JavaScript XML), which looks similar to HTML but is compiled into React elements.
4 JSX:
- JSX allows you to write declarative views in React components.
- Always wrap JSX elements in a single parent element (usually a
<div>
or a React fragment<>...</>
). - Use curly braces
{}
to embed JavaScript expressions inside JSX.
5 State and Props:
- Props are the data passed from a parent component to a child component.
- State is used for managing local component data that can change over time.
- Avoid modifying state directly; use
setState()
for class components anduseState()
hook for functional components.
6 Lifecycle methods (for class components):
- React class components have lifecycle methods like
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
. - However, with React 16.3 and above, functional components can also use lifecycle methods through React hooks.
7 React Hooks:
- Hooks allow you to use state and other React features in functional components.
- Commonly used hooks are
useState
,useEffect
,useContext
,useReducer
, etc. - Follow the Rules of Hooks, ensuring hooks are only called at the top level of the functional component.
8 Handling Events:
- React uses camelCase event naming convention (e.g.,
onClick
,onChange
) instead of lowercase HTML attributes. - Bind event handlers to the appropriate components or use arrow functions to avoid issues with
this
context.
9 Conditional Rendering:
Use if
statements or ternary operators within JSX to conditionally render components or elements.
10 Styling:
- Apply CSS classes to components for styling.
- Consider using CSS-in-JS libraries like styled-components or CSS modules for a more scoped approach.
11 Routing (for SPAs):
Use react-router or similar libraries for managing client-side routing in single-page applications.
12 State Management (for complex apps):
For larger applications, consider using state management libraries like Redux or MobX to handle global state.
13 Testing:
Write unit tests for your components using testing libraries like Jest and React Testing Library.
14 Performance:
- Optimize performance using React.memo, useCallback, useMemo, and other techniques when necessary.
- Use the React DevTools to analyze component rendering and performance.
15 Learning Resources:
- Official React documentation: https://reactjs.org/
- React hooks documentation: https://reactjs.org/docs/hooks-intro.html
- Community-driven tutorials and articles on sites like Medium, Dev.to, and YouTube.
Remember, React.js is a powerful and ever-evolving library, so stay updated with the latest best practices and changes in the React ecosystem. Happy coding!