All about Mongoose Connections
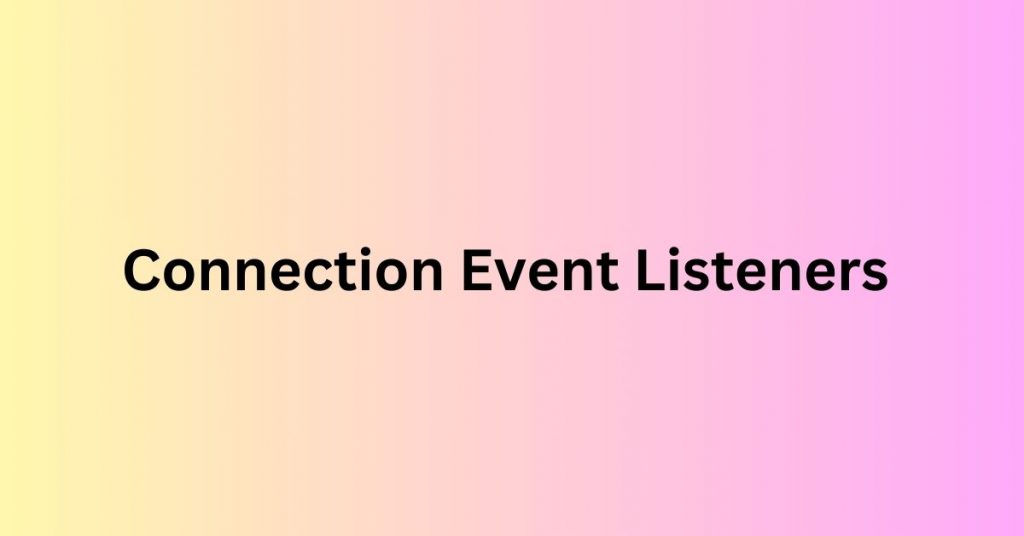
Handling Connection Events play a great role to know condition of database Connections. So try to use these kinds of Connections Events. It is a good practice to handle connection events to know when the connection is open, connected, close or if there is any error. You can add event listener for this purpose.
import mongoose from "mongoose";
export async function connect() {
try {
mongoose.connect(process.env.MONGO_URI!)
const connection = mongoose.connection
connection.on("connected", () => {
console.log("database connected")
})
connection.on("error", (err) => {
console.log("Mongodb connection error, make sure db is up and running" + err)
process.exit()
})
} catch (error) {
console.log(error)
console.log("something went wront in database")
}
}
There are so many connections available. If you are using mongoose.connect(), you can use the following Event Listeners.
mongoose.connection.on('connected', () => console.log('connected'));
mongoose.connection.on('open', () => console.log('open'));
mongoose.connection.on('disconnected', () => console.log('disconnected'));
mongoose.connection.on('reconnected', () => console.log('reconnected'));
mongoose.connection.on('disconnecting', () => console.log('disconnecting'));
mongoose.connection.on('close', () => console.log('close'));
If you want to know more about things , you can follow following website….#