What is the difference between Navigate Component and useNavigate hook???
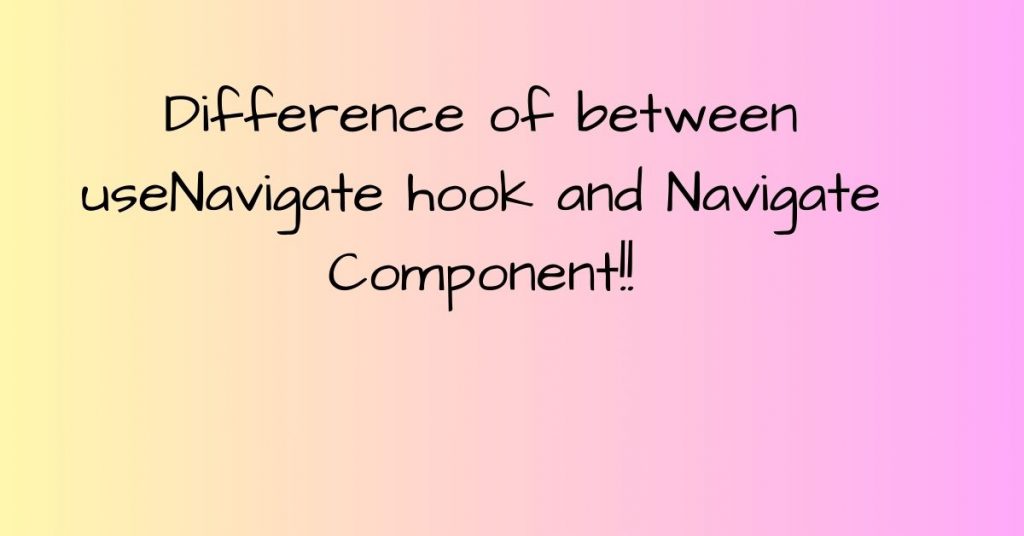
useNavigate
and Navigate
serve similar purposes in terms of navigation within a React application using React Router, but they are used in different contexts and have some key differences.
useNavigate:
useNavigate
is a hook provided by React Router for functional components.- It returns a navigate function that you can use to programmatically navigate to different routes.
- It is typically used inside functional components to get access to the navigation function.
import { useNavigate } from 'react-router-dom'; function MyComponent() { const navigate = useNavigate(); const handleClick = () => { navigate('/another-route'); }; return ( <button onClick={handleClick}>Go to another route</button> ); }
Navigate component:
Navigate
is a React component provided by React Router for use within the JSX of your components.- It is used for declarative navigation, and you include it in the JSX structure of your component based on certain conditions.
- It’s often used when you want to conditionally navigate based on some logic.
import { Navigate } from 'react-router-dom';
function PrivateRoute({children }) {
const token = window.localStorage.getItem("token");
return token ? <>{children}</> : <Navigate to="/" ;
}
In summary, useNavigate
is a hook used to get the navigation function in functional components, while the Navigate
component is used for declarative navigation within the JSX of your components. Both are tools provided by React Router to handle navigation in a React application, and the choice between them depends on your specific use case and whether you prefer imperative or declarative navigation.